Introduction
What is JavaScript?
JavaScript is a very powerful client-side scripting language. JavaScript is used mainly for enhancing the interaction of a user with the webpage. In other words, you can make your webpage more lively and interactive, with the help of JavaScript. JavaScript is also being used widely in game development and Mobile application development.
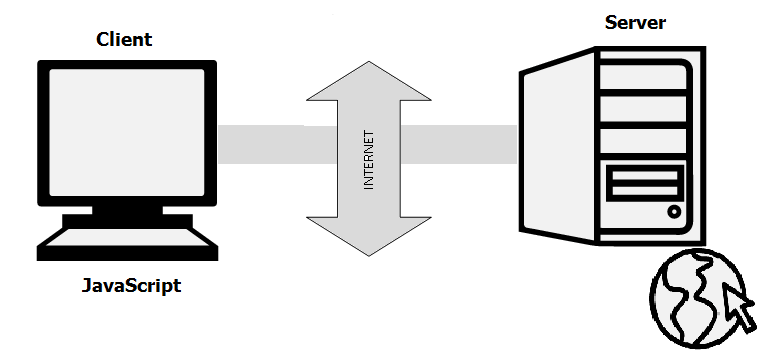
Javascript History
JavaScript was developed by Brendan Eich in 1995, which appeared in Netscape, a popular browser of that time.
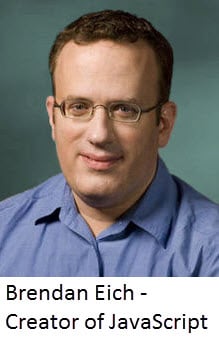
The language was initially called LiveScript and was later renamed JavaScript. There are many programmers who think that JavaScript and java are the same. In fact, JavaScript and Java are very much unrelated. Java is a very complex programming language whereas JavaScript is only a scripting language. The syntax of JavaScript is mostly influenced by the programming language C.
How to Run JavaScript?
Being a scripting language, JavaScript cannot run on its own. In fact, the browser is responsible for running JavaScript code. When a user requests an HTML page with JavaScript in it, the script is sent to the browser and it is up to the browser to execute it. The main advantage of JavaScript is that all modern web browsers support JavaScript. So, you do not have to worry about whether your site visitor uses Internet Explorer, Google Chrome, Firefox or any other browser. JavaScript will be supported. Also, JavaScript runs on any operating system including Windows, Linux or Mac. Thus, JavaScript overcomes the main disadvantages of VBScript (Now deprecated) which is limited to just IE and Windows.
Tools You Need
To start with, you need a text editor to write your code and a browser to display the web pages you develop. You can use a text editor of your choice including Notepad++, Visual Studio Code, Sublime Text, Atom or any other text editor you are comfortable with. You can use any web browser including Google Chrome, Firefox, Microsoft Edge, Internet Explorer etc.
JavaScript and Java
JavaScript and Java are similar in some ways but fundamentally different in some others. The JavaScript language resembles Java but does not have Java’s static typing and strong type checking. JavaScript follows most Java expression syntax, naming conventions and basic control-flow constructs which was the reason why it was renamed from LiveScript to JavaScript.
In contrast to Java’s compile-time system of classes built by declarations, JavaScript supports a runtime system based on a small number of data types representing numeric, Boolean, and string values. JavaScript has a prototype-based object model instead of the more common class-based object model. The prototype-based model provides dynamic inheritance; that is, what is inherited can vary for individual objects. JavaScript also supports functions without any special declarative requirements. Functions can be properties of objects, executing as loosely typed methods.
JavaScript is a very free-form language compared to Java. You do not have to declare all variables, classes, and methods. You do not have to be concerned with whether methods are public, private, or protected, and you do not have to implement interfaces. Variables, parameters, and function return types are not explicitly typed.
Java is a class-based programming language designed for fast execution and type safety. Type safety means, for instance, that you can’t cast a Java integer into an object reference or access private memory by corrupting Java bytecodes. Java’s class-based model means that programs consist exclusively of classes and their methods. Java’s class inheritance and strong typing generally require tightly coupled object hierarchies. These requirements make Java programming more complex than JavaScript programming.
In contrast, JavaScript descends in spirit from a line of smaller, dynamically typed languages such as HyperTalk and dBASE. These scripting languages offer programming tools to a much wider audience because of their easier syntax, specialized built-in functionality, and minimal requirements for object creation.
JavaScript | Java |
---|---|
Object-oriented. No distinction between types of objects. Inheritance is through the prototype mechanism, and properties and methods can be added to any object dynamically. | Class-based. Objects are divided into classes and instances with all inheritance through the class hierarchy. Classes and instances cannot have properties or methods added dynamically. |
Variable data types are not declared (dynamic typing, loosely typed). | Variable data types must be declared (static typing, strongly typed). |
Cannot automatically write to hard disk. | Can automatically write to hard disk. |
For more information on the differences between JavaScript and Java.
JavaScript and the ECMAScript specification
JavaScript is standardized at Ecma International— the European association for standardizing information and communication systems (ECMA was formerly an acronym for the European Computer Manufacturers Association) to deliver a standardized, international programming language based on JavaScript. This standardized version of JavaScript, called ECMAScript, behaves the same way in all applications that support the standard. Companies can use the open standard language to develop their implementation of JavaScript. The ECMAScript standard is documented in the ECMA-262 specification. See New in javaScript to learn more about different versions of JavaScript and ECMAScript specification editions.
The ECMA-262 standard is also approved by the ISO (International Organization for Standardization) as ISO-16262. You can also find the specification on the the Ecma International website. The ECMAScript specification does not describe the Document Object Model (DOM), which is standardized by the World Wide Web Consortium (W3C) and/or WHATWG (Web Hypertext Application Technology Working Group). The DOM defines the way in which HTML document objects are exposed to your script. To get a better idea about the different technologies that are used when programming with JavaScript.
JavaScript documentation versus the ECMAScript specification
The ECMAScript specification is a set of requirements for implementing ECMAScript. It is useful if you want to implement standards-compliant language features in your ECMAScript implementation or engine (such as SpiderMonkey in Firefox, or V8 in Chrome).
The ECMAScript document is not intended to help script programmers. Use the JavaScript documentation for information when writing scripts.
The ECMAScript specification uses terminology and syntax that may be unfamiliar to a JavaScript programmer. Although the description of the language may differ in ECMAScript, the language itself remains the same. JavaScript supports all functionality outlined in the ECMAScript specification.
The JavaScript documentation describes aspects of the language that are appropriate for a JavaScript programmer.
Getting started with JavaScript
Getting started with JavaScript is easy: all you need is a modern Web browser. This guide includes some JavaScript features which are only currently available in the latest versions of Firefox, so using the most recent version of Firefox is recommended.
The Web Console tool built into Firefox is useful for experimenting with JavaScript; you can use it into two modes: single-line input mode, and multi-line input mode.
Single-line input in the Web Console
The Web Console shows you information about the currently loaded Web page, and also includes a JavaScript interpreter that you can use to execute JavaScript expressions in the current page.
To open the Web Console (Ctrl+Shift+I on Windows and Linux or Cmd–Option–K on Mac), open the Tools menu in Firefox, and select “Developer ▶ Web Console“.
The Web Console appears at the bottom of the browser window. Along the bottom of the console is an input line that you can use to enter JavaScript, and the output appears in the panel above:

The console works the exact same way as eval
: the last expression entered is returned. For the sake of simplicity, it can be imagined that every time something is entered into the console, it is actually surrounded by console.log
around eval
, like so:
function greetMe(yourName) {
alert("Hello " + yourName)
}
console.log(eval('3 + 5'))
Multi-line input in the Web Console
The single-line input mode of the Web Console is great for quick testing of JavaScript expressions, but although you can execute multiple lines, it’s not very convenient for that. For more complex JavaScript, you can use the multi-line line input mode.
Hello world
To get started with writing JavaScript, open the Web Console in multi-line mode, and write your first “Hello world” JavaScript code:
(function(){
"use strict";
/* Start of your code */
function greetMe(yourName) {
alert('Hello ' + yourName);
}
greetMe('World');
/* End of your code */
})();
Press Cmd+Enter or Ctrl+Enter (or click the Run button) to watch it unfold in your browser!
In the following pages, this guide introduces you to the JavaScript syntax and language features, so that you will be able to write more complex applications.
But for now, remember to always include the (function(){"use strict";
before your code, and add })();
to the end of your code. You will learn what these mean, but for now they can be thought of as doing the following:
1. Massively improve performance.
2. Prevent stupid semantics in JavaScript that trip up beginners.
3. Prevent code snippets executed in the console from interacting with one-another (e.g., having something created in one console execution being used for a different console execution).
How to Install JavaScript In Internet Explorer?
- On the web browser, click on Tools or the “Tools” icon which looks like a gear (generally present on the right side) and select Internet Options.
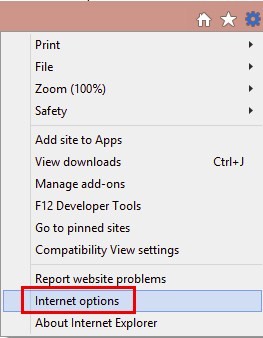
- Then the “Internet Options” window opens, there we need to select the Security tab.
- On selecting the “Security” tab, we need to select Internet zone and then click on the “Custom level…” button.
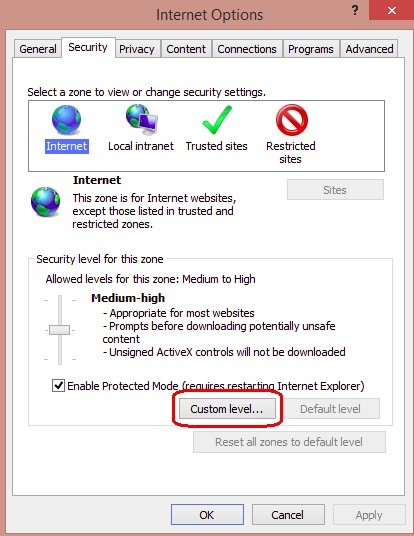
- After clicking on the custom level… a pop-up box Security Settings-Internet Zone will appear, there we need to go to scripting section and change it to enable.
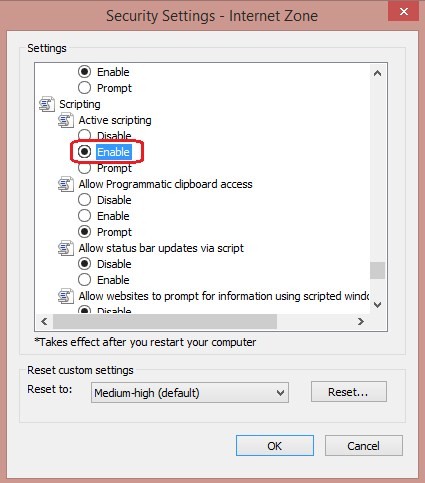
- Now we will get a “Warning!” window popped up asking, “Are you sure you want to change the settings for this zone?” select “yes”.
- Click “ok” at the bottom of the Internet Options and all other windows open to close them.
These are the steps to install JavaScript in IE (Internet Explorer). Now as we have installed JavaScript we need an editor to write a script. So now we will see how to install JavaScript editor PyCharm, where we can write JavaScript. Pycharm is a cross-platform editor, here we will be using it for JavaScripts.
These are the steps to install JavaScript in IE (Internet Explorer). Now as we have installed JavaScript we need an editor to write a script. So now we will see how to install JavaScript editor PyCharm, where we can write JavaScript. Pycharm is a cross-platform editor, here we will be using it for JavaScripts.
PyCharm Supports Which languages?
PyCharm can be used for development in many languages. JavaScript is also one of the languages supported by PyCharm. Using professional edition we can develop Django, Flask and Pyramid applications as well. Also, it fully supports HTML5, CSS and XML. All the languages are bundled in the IDE via plugins, and they are present by default. We can add more plugins so that it can support other languages and frameworks also. For this to achieve we need to add more plugins and we need to set them up when we launch the first IDE.
How to Install JavaScript Editor PyCharm?
Let us discuss the steps required to install JavaScript Editor PyCharm.
- We need to download PyCharm from the below link for the Community.
https://www.jetbrains.com/pycharm/download/
- Once the exe file is downloaded, run the exe to install PyCharm.
- Once we run the exe, setup wizard starts. Click “Next”.
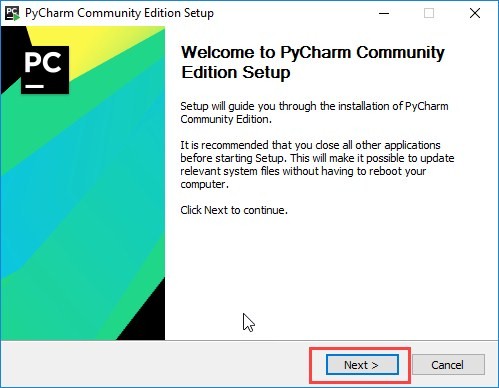
- Now on the next screen, we need to verify the installation path or change if required. Now we need to Click “Next”.
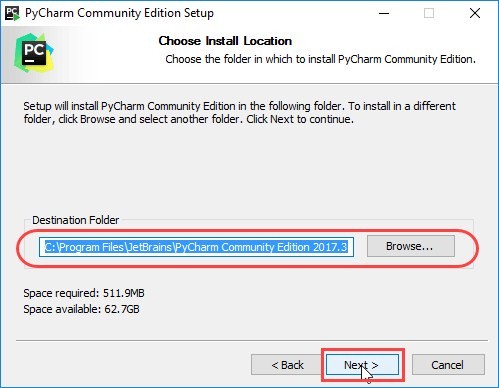
- Next screen gives you an option to create a desktop shortcut if you want. Again we need to Click “Next”.
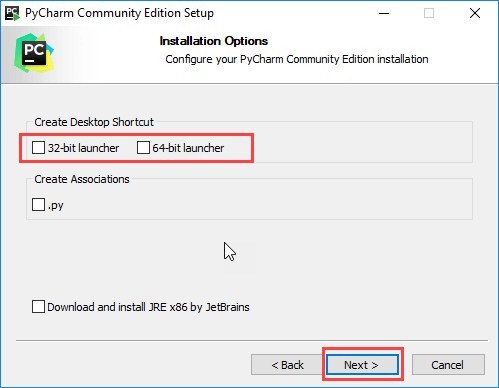
- In this step, we need to give a folder where we need to save our programs. And then Click “Install”.
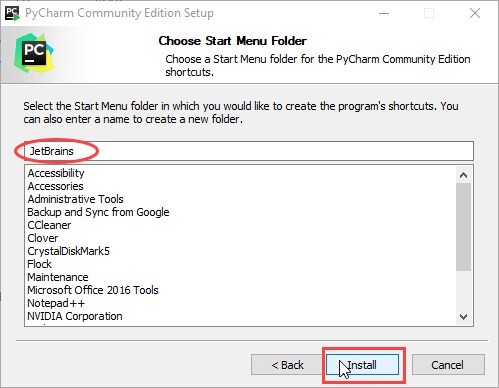
- Now the installation should be in progress.
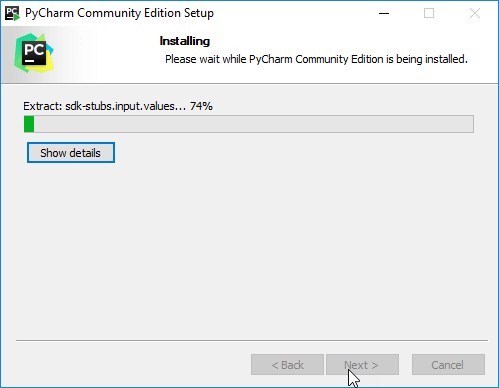
- Once the installation is finished, we will get a pop-up box where it will show a message that PyCharm is installed. If we want to launch it we need to check the box “Run PyCharm Community Edition” box first and then click “Finish”.
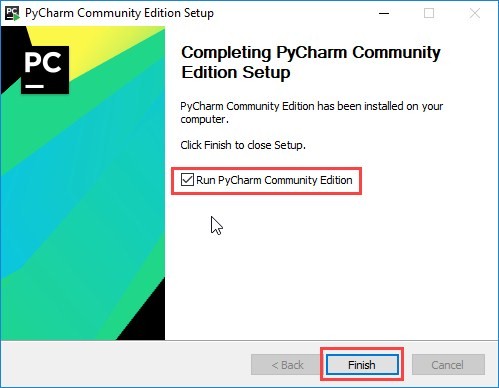
- Once we check the box and click the finish we will get the following window.
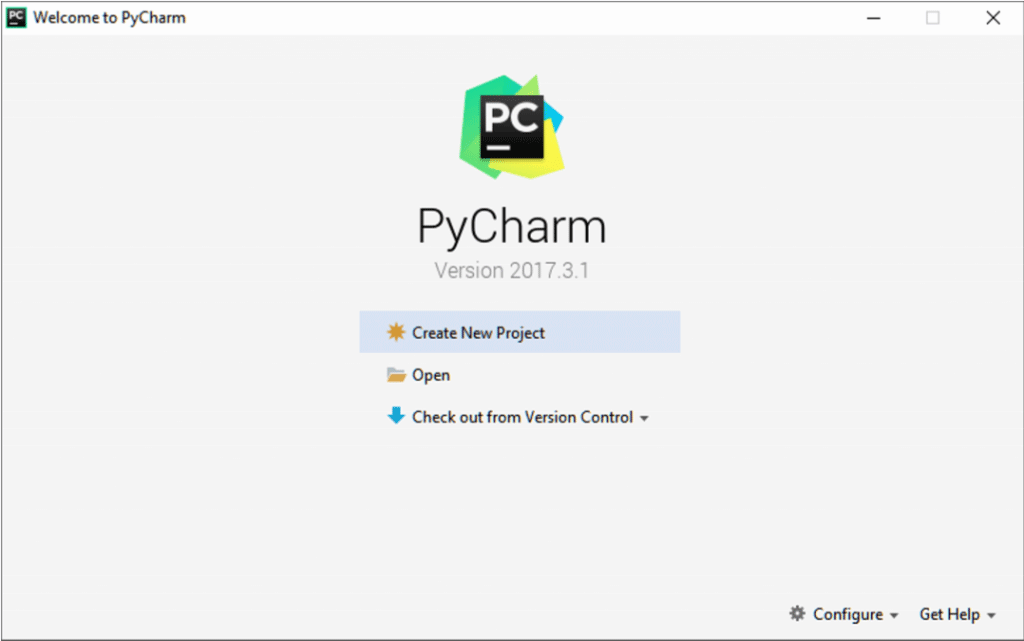
This means the installation is successfully completed. Now we can use this editor for our program development.
To Run a file with JavaScript from PyCharm
- In the editor, we need to open the HTML file with the Javascript reference. This HTML file does necessarily have to be the one that implements the starting page of the application.
- Do one of the following:
- Choose View | Open in Browser on the main menu or press Alt+F2. Then select the desired browser from the pop-up menu.
- We need to move our mouse pointer over the code to show the browser icons bar:
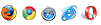
Click the icon that indicates the desired browser.
Conclusion
- JavaScript is a scripting language which does not need to be compiled. We can use JavaScript for storing information, games, and security.
- Here we have seen the steps to Install JavaScript.
- PyCharm is the editor used for writing scripts. Here we have shown the steps to install it and how it works.