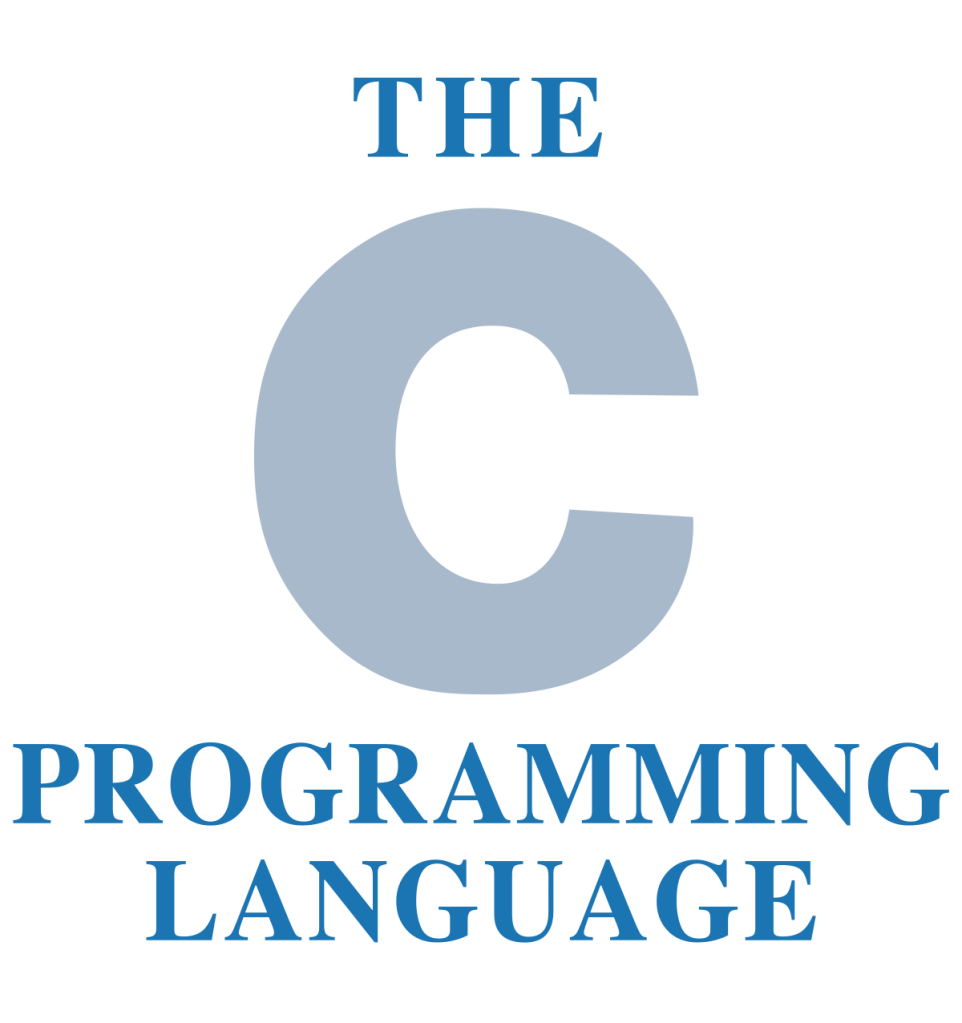
Introduction
C is a procedural programming language. It was initially developed by Dennis Ritchie in the year 1972. It was mainly developed as a system programming language to write an operating system. The main features of C language include low-level access to memory, a simple set of keywords, and clean style, these features make C language suitable for system programmings like an operating system or compiler development.
Many later languages have borrowed syntax/features directly or indirectly from C language. Like syntax of Java, PHP, JavaScript, and many other languages are mainly based on C language. C++ is nearly a superset of C language (There are few programs that may compile in C, but not in C++).
Beginning with C programming:
- Structure of a C program
After the above discussion, we can formally assess the structure of a C program. By structure, it is meant that any program can be written in this structure only. Writing a C program in any other structure will hence lead to a Compilation Error.The structure of a C program is as follows:
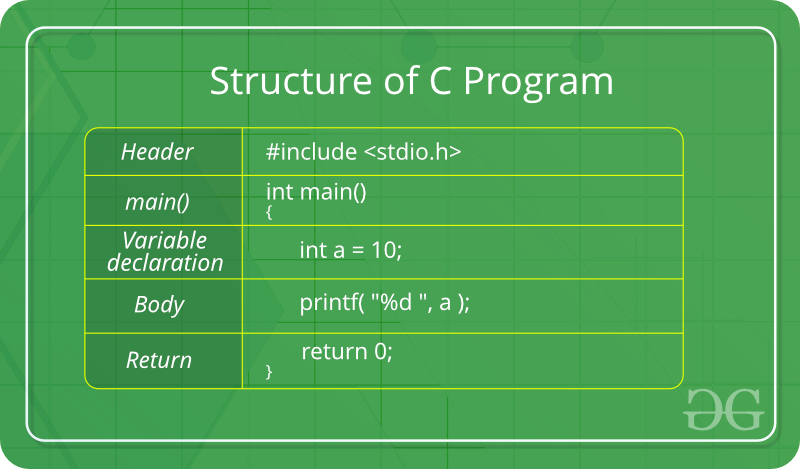
The components of the above structure are:
- Header Files Inclusion: The first and foremost component is the inclusion of the Header files in a C program.
A header file is a file with extension .h which contains C function declarations and macro definitions to be shared between several source files.Some of C Header files:- stddef.h – Defines several useful types and macros.
- stdint.h – Defines exact width integer types.
- stdio.h – Defines core input and output functions
- stdlib.h – Defines numeric conversion functions, pseudo-random network generator, memory allocation
- string.h – Defines string handling functions
- math.h – Defines common mathematical functions
Syntax to include a header file in C:
#include
2. Main Method Declaration: The next part of a C program is to declare the main() function. The syntax to declare the main function is:
Syntax to Declare main method:
int main()
{}
3. Variable Declaration: The next part of any C program is the variable declaration. It refers to the variables that are to be used in the function. Please note that in the C program, no variable can be used without being declared. Also in a C program, the variables are to be declared before any operation in the function.
Example:
int main()
{
int a;
.
.
4. Body: Body of a function in C program, refers to the operations that are performed in the functions. It can be anything like manipulations, searching, sorting, printing, etc.
Example:
int main()
{
int a;
printf("%d", a);
.
.
5. Return Statement: The last part in any C program is the return statement. The return statement refers to the returning of the values from a function. This return statement and return value depend upon the return type of the function. For example, if the return type is void, then there will be no return statement. In any other case, there will be a return statement and the return value will be of the type of the specified return type.
Example:
int main()
{
int a;
printf("%d", a);
return 0;
}
2. Writing first program:
Following is first program in C
#include <stdio.h> int main(void) {Writing first program: |
- Let us analyze the program line by line.
Line 1: [ #include <stdio.h> ] In a C program, all lines that start with # are processed by Preprocessor which is a program invoked by the compiler. In a very basic term, Preprocessor takes a C program and produces another C program. The produced program has no lines starting with #, all such lines are processed by the preprocessor. In the above example, preprocessor copies the preprocessed code of stdio.h to our file. The .h files are called header files in C. These header files generally contain declaration of functions. We need stdio.h for the function printf() used in the program.Line 2 [ int main(void) ] There must to be starting point from where execution of compiled C program begins. In C, the execution typically begins with first line of main(). The void written in brackets indicates that the main doesn’t take any parameter (See this for more details). main() can be written to take parameters also. We will be covering that in future posts.
The int written before main indicates return type of main(). The value returned by main indicates status of program termination. See this post for more details on return type.Line 3 and 6: [ { and } ] In C language, a pair of curly brackets define a scope and mainly used in functions and control statements like if, else, loops. All functions must start and end with curly brackets.Line 4 [ printf(“shenky”); ] printf() is a standard library function to print something on standard output. The semicolon at the end of printf indicates line termination. In C, semicolon is always used to indicate end of statement.Line 5 [ return 0; ] The return statement returns the value from main(). The returned value may be used by operating system to know termination status of your program. The value 0 typically means successful termination. - How to excecute the above program:
Inorder to execute the above program, we need to have a compiler to compile and run our programs. There are certain online compilers like
that can be used to start C without installing a compiler.
How to install Turbo C in Windows 7/8
Installation of Turbo C on Windows 7/8:
Turbo C++ 3.0 is the next version to Turbo C 2.0 used to execute both C and C++ programs. It is not compatible with the latest versions of Windows 7 and 8. Though it runs on Windows 7 32 bit version, may not be loaded in full screen mode (loads in small window). It doesn’t run at all on Windows 7/8 64bit versions because 16bit DOS sub system is not available on Windows 7/8.
Though number of IDEs are available for Windows 7/8, most of the colleges teach C programming on the Turbo C IDE due to its simplicity, speed and low cost.
We can make run the Turbo C IDE on any version of Windows may it be 32bit or 64bit using a utility software called DOSBOX.
All the process is automated in a single executable setup file. You can install both the DOSBOX and Turbo C++ 3.0 in it, by following the setup wizard.
Step1: Click the following download link to download “turboC_C++.zip”
Step2: Unzip the zip file “turboC_C++.zip” and double click on “TurboC_C++” executable file and follow setup wizard.
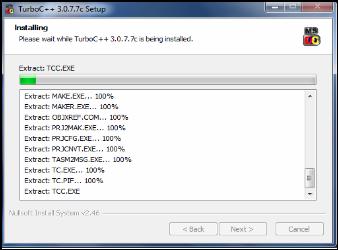
- Select “next”
- Accept agreement by checking “I accept the terms of license agreement”
- Select “install”
- Select “finish”
Step3: Double click on the TurboC++ icon which is on desktop to start Turbo C IDE.

Step4: Write any C program and execute as if you are using IDE on Windows XP
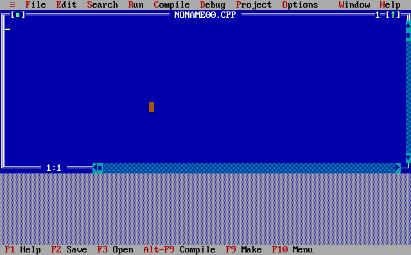
Step5: Here we will come out of DOSBOX and into DOS shell on selecting quitting the IDE (File-Quit)
Use exit command to comedown to the windows environment

Installation of TURBO C compiler on Windows XP/vista:
Turbo C is an integrated development environment and compiler for C programming language from Borland. It was first introduces in 1987 as Turbo C 1.0. The second version of Turbo C 2.0 was released during late 1989. In 1990 Borland replaced Turbo C with Turbo C++ 3.0. The latest version is Turbo moniker released in 2006.
Though Turbo C compiler may not be used in commercial development, it is best to startup with, Due to its simple, speed and user friendly environment.
Here we will see the step by step procedure of installation and configuration of Turbo C++ 3.0 under windows XP. It can be used to write and compile both C and C++ programs.
Installation procedure of Turbo C on Windows XP/Vista:
Step1: click the following download link to download “tc3_setup.zip”
Step2: Unzip the “tc3_setup.zip” and double click on “tc3_setup” file

Step3: Select the “install” button

Step4: Select close to complete installation

Configuring Turbo C:
Once the installation process is completed then we need to specify the locations of include, lib, output and source directories to turbo C
Step1: Create an output directory any where on the hard disk. All the object files and executable files generated by the compiler would be stored in the output directory.
Here, i am creating my output directory as c:\tc\works

Step2: Open the C-IDE (Integrated Development Environment) by double click on “Turbo C++ 3.0” which is on desktop.

Step3: Specify the locations of include, lib, source and output folders in the directories option
On Authors machine Directories are
Include Directory : c:\tc\include
Lib Directory : c:\tc\lib
Output Directory: c:\tc\works
Source Directory: c:\tc
Select Options (Alt+O), Directories (D)

These details may change version to version, hoping you will set according to your machine
Step4: Save the options
We have to save the options whenever we modify the options otherwise changed settings may not come into action. To save the options,
Select Options(Alt+O), Save (S)

Executing a C program in Turbo C:
1. Open the C-IDE (Integrated Development Environment) by double click on “Turbo C++ 3.0” which is on desktop.

2. To create a new file select File (Alt+F), New (N) [F is the hot key to File option and N is the hot key to New].

3. Type the program.
1234567 | #include<stdio.h> int main() { printf ( "Hello World" ); printf ( "This is my first program.." ); return 0; } |
4. Save the program by selecting File (Alt+F), Save (S) or select F2 (It is the short-cut to save option)
Here the name of C program must be saved with .c extension. (Otherwise takes .cpp as the default file extension)


5.Execute the program by pressing Ctrl+F9.
6. View the output by pressing Alt+F5.

Output of second execution

8. Maximizing the Turbo C IDE window size (Full screen)
- Right click on the tool bar of Turbo C IDE
- Select properties
- Select “Full Screen” option under “Display Options”.
- Select OK


Short – cut to Maximize and minimize the Turbo C window is Alt + Enter
Some modifications to the program structure:
The output of first execution is still there, even after the completion of second execution. It may be confusing output while experimenting with the program. We can use “clrscr();” to clear the output generated by previous execution. We can also use “getch();“ to immediately display the output instead of using Alt+F5 to view the output.
In Turbo C writing #include<stdio.h> at the beginning of program is not mandatory to use printf(), scanf(), clrscr() and getch() because these are the default functions of Turbo C compiler.
Modified structure of C program (Turbo C only):

Tools to execute a C program
BY shanky
Tools to execute a C program:
The code written in C language is called the source code, which is saved with .c file extension (hello.c). We need some tools to debug, compile and execute a C program. These are
- Debugger
- Preprocessor
- Compiler
- Linker
Meaning of bug is an error. Debugger is a tool takes the source code written in C language and reports errors if any. It helps to rectify the errors in the program.
Preprocessor is a tool which performs some modifications to the source code and sends the modified code to the compiler.
The compiler is an important tool take the source code and produces object code. The object code is saved with .o or .obj file extension with the original name of C program (hello.o or hello.obj). There are different compilers available to compile a C program. Some of these are gcc (GNU Compiler Collection), TURBO C, Quick C, Microsoft C, Aztech C, Zortech C, Lattice C, Watcom C, Green Leaf C and Vitamin C etc.
Linker is a tool includes files from the runtime support library to the object code and generates native executable code, which is saved with .exe file extension (hello.exe).
To successfully debug and execute a C program we do need all these tools.

C IDE (Integrated Development Environment):
As discoursed earlier, we need an editor, debugger, pre-processor, compiler, linker, runtime support library to write and execute any C program. All these tools are integrated with in a single software called IDE.

Now we will see installation and configuration of different IDEs and compilers under different platforms. Skip to programming if you are already using an IDE.
In this course we are going to discourse
- TURBOC Installation on windows XP
- TURBOC installation and configuration on 64bit platforms like Windows7/Windows8
- Execution of a C program on Linux platform
- Execution of a C program using gcc compiler (GNU compiler collection)
- Installation and configuration of gcc for commercial editors like edit+, notepad++ and eclipse on windows.